1.3 Object Assignment
In the last section, you have seen the power of R as a fancy calculator. However, in order to do more complicated and interesting tasks, it is often helpful to store intermediate results for future use.
Let’s take a look at a concrete example. Say if you want to do the following three calculations, all involving exp(3) / log(20,3) * 7
.
(exp(3)/log(20, 3) * 7) + 3 #addition
(exp(3)/log(20, 3) * 7) - 3 #subtraction
(exp(3)/log(20, 3) * 7)/3 #division
Here, you need to type the same expression exp(3) / log(20,3) * 7
three times, which is a bit cumbersome. In this section, you will learn how to do object assignment, which avoid redundancy and make your code more concise.
1.3.1 What is an R Object?
Before delving into the details, let’s first introduce the concept of object, which is perhaps the most fundamental thing in R. In principle, everything that exists in R is an object. For example, the number 5
is an object, the expression 1 + 2
is an object, and the expression exp(3) / log(20,3) * 7
is also an object.
If you run 5
, you will get one element of value 5 from the output. Similarly, if you run 1 + 2
, you will get one element of value 3 from the output. You can try to run exp(3) / log(20,3) * 7
by yourself. In these three examples, you can see that there is only one element in each object.
However, an object can contain more than one elements, and each element has its own value, which is possibly different from that of another element. Naturally, different objects can contain different values. In the following sections, you will learn how to create objects and assign values to them.
1.3.2 Assignment Operation with <-
With the importance of objects in mind, let’s learn how to do object assignments in R. To do object assignments, you need to assign value(s) to a name via the assignment operator, which will create a new object with the name you specified. Once the object assignment operation is done, you can simply use the name in subsequent calculations without redundancy. Let’s start with a simple example, assigning the value 5 to the name x_num
.
The assignment operation has three components. From left to right,they are:
- the first component
x_num
is the object name of a new object, which has certain naming rules that will be discussed shortly in Section 1.3.3. - The second component is the assignment operator
<-
, which is a combination of the less than sign<
immediately followed by the minus sign-
, with no space in between. - The final component is the object name’s assigned value(s), which is 5 here.
There is no space between <
and -
in the assignment operator <-
. Note that although =
may also appear to be working as the assignment operator, it is not recommended as =
is usually reserved for specifying the value(s) of arguments in a function call, which will be introduced in Section 2.1.
After running the code above, you will see no output in the console, unlike the situation when we ran 1 + 2
which gives us the answer 3
(as shown in Figure 1.18). You may be wondering, did we successfully make our first assignment operation?
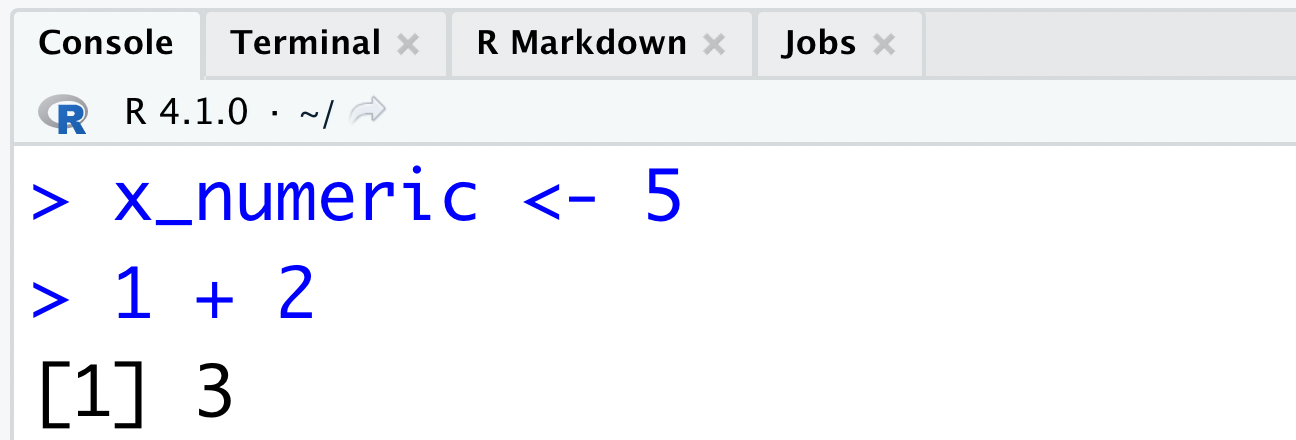
Figure 1.18: No output
To verify it, you can run the code with just the object name to check its value. (For all named objects, you can get their value(s) by running codes with just their names.)
Great! The output is 5, indicating that you have successfully assigned the value 5 to the name x_num, and you have created a new object x_num
. From now on, you can use x_num
instead of 5
to do the subsequent calculations because x_num
and 5
have the same value.
Note that R object names are case-sensitive. For example, you have defined x_num
, but if you type X_num
, the console will return an error message as follow.
In addition, you can assign value(s) of an expression to a name. Let’s try to simplify the three expressions we showed at the beginning of this section. It is easy to observe that the three expressions share a common term exp(3) / log(20,3) * 7
. Let’s assign the common term to a name.
Now you have successfully created an object y_num
with value 51.561191. Using the named object y_num
, you can simplify the three calculations as follows.
Note that in the object assignment process, it is not the expression itself but rather the value(s) of the expression, that is assigned to a name. So you will not get the expression exp(3) / log(20,3) * 7
by running y_num
.
You can also try the following examples by yourself.
Clearly, using the object assignment, you can greatly simplify the code and avoid redundancy.
1.3.3 Object naming rule
As you now see, the assignment operation in R is very straightforward. In general, R is very flexible in the name you can give to an object. However, there are three important rules you need to follow.
a. Must start with a letter or . (period)
In addition, if starting with period, the second character can’t be a number.
b. Can only contain letters, numbers, _
(underscore), and .
(period)
One recommended naming style is to use lowercase letters and numbers, and use underscore to separate words within a name. So you can use relatively longer names that is more readable. For example, this_is_name_6
and super_rich_88
are great names.
c. Can not use special keywords as names.
For example, TRUE <- 12
is not permitted as TRUE
is a special keyword in R. You can see from the following that this assignment operation leads to an error message.
Some commonly used reserved keywords that cannot be used as names are listed as below.
TRUE | else |
FALSE | for |
NA | while |
Inf | break |
NaN | next |
function | repeat |
if | return |
To get a complete list of reserved words, you can run the following code.
1.3.4 Review objects in environment
At this point, we’ve introduced the rules of creating objects in R. Now, you can also confirm the success of object assignments by inspecting the Environment, located in the top right panel (panel3 in Figure 1.4 in Section 1.1).
If you exercised previous examples, you can find the newly assigned objects x_num
and y_num
(possibly also z_num1
and z_num2
) in your Environment Viewer. You may also notice that the name TRUE
, which we tried but failed to assign the value 12 to, doesn’t appear in the Environment (as shown in Figure 1.19). You can check all the named objects and their values in this area.
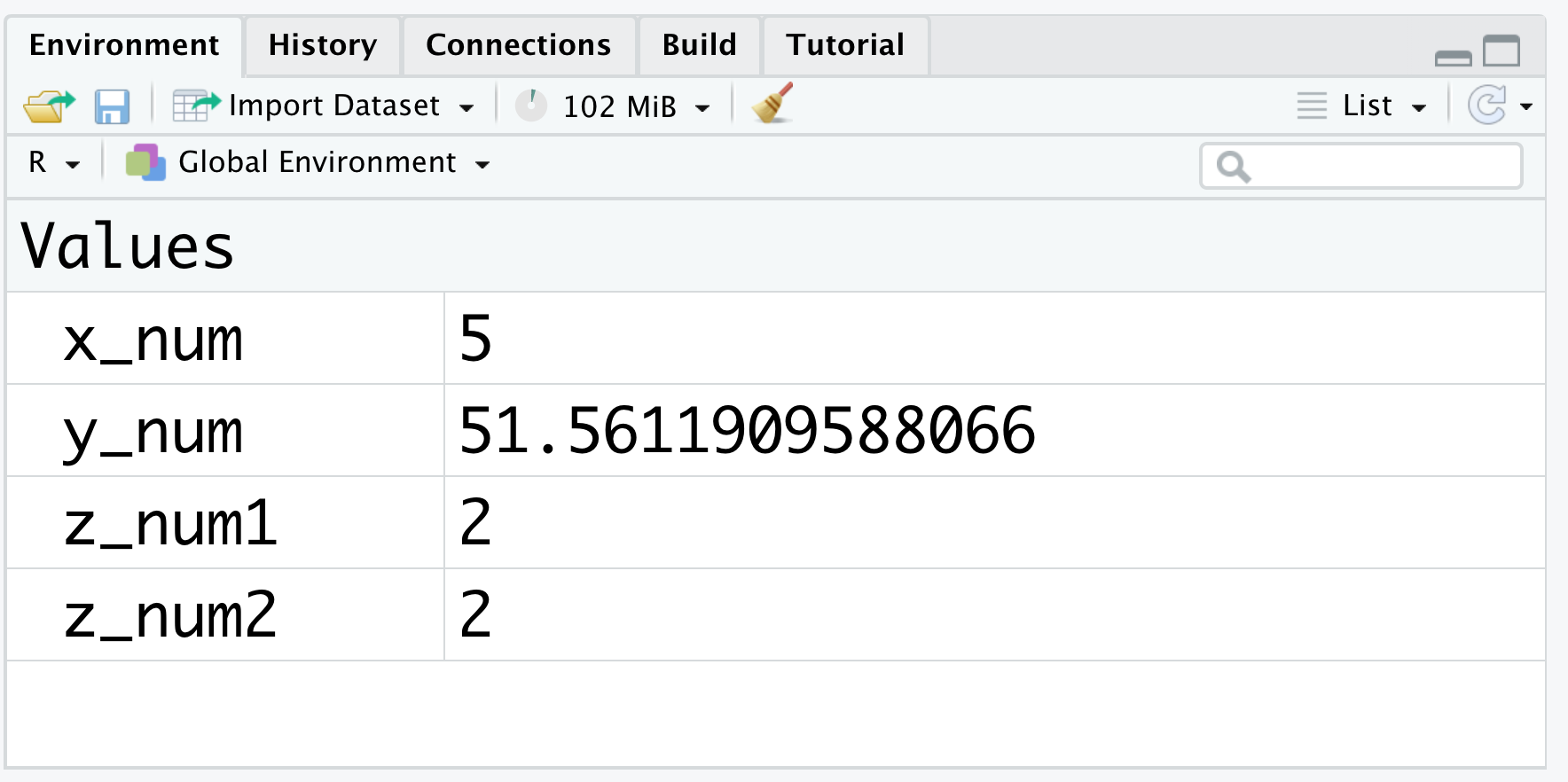
Figure 1.19: The environment (I)
From the picture above, you can see that the value of x_num
is 5. In this case, let’s try to assign the new value 6 to x_num
and see what will happen next.
Now you can see that the value of x_num
has changed from 5 to 6. Generally, when assigning a new value to an object, R will update the object’s value, and the previous value will no longer be stored. You can verify the result by inspecting the Environment tab, where only the new value of the object will be displayed.
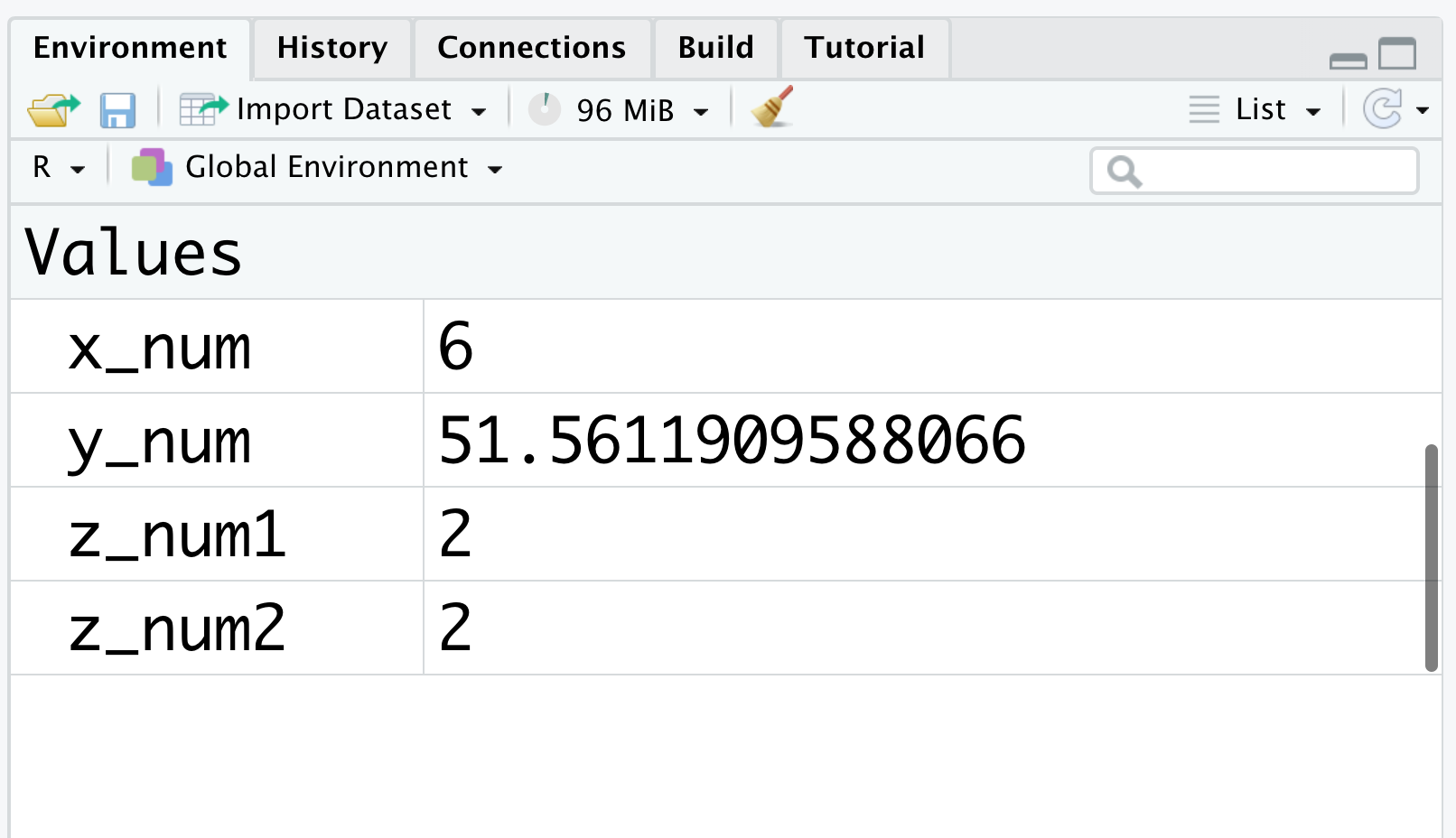
Figure 1.20: The environment (II)
So it is helpful to monitor the environment from time to time to make sure everything looks fine. Notice that objects without names will not be shown in the environment.
You can also see the list of all the named objects (just names without values) using the built-in R function ls()
.
All the objects shown in the environment or on the list are stored in the memory, so they are available for us in subsequent codes. It is a good habit to do object assignments if you want to retrieve their values at a later time.
1.3.5 Object types
So far in this section, you have learned how to do object assignments. The values you assigned are all numbers, i.e. of numeric type. Actually, an object may contain more than one values. Also, an object may contain values other than the numeric type, such like character and logical ones. Depending on the composition of values, the object belongs to one particular type.
Type | Section |
---|---|
Atomic Vector | 2 |
Matrix | 3.1 |
Array | 3.2 |
Data Frame | 3.3 |
Tibble | 3.4 |
List | 3.5 |
We will focus on atomic vectors in Chapter 2 and discuss other object types in Chapter 3.
While some of the object types look more intuitive than others, you have nothing to worry about since we have the next two chapters devoted to the details of R objects. Objects are the building blocks of R programming and it will be time well spent mastering every object type.